make this a bit shorter
To design a simulation to generate frequencies for the probability of a client choosing model B with a car garage, we can follow these steps:
1. Define the probability of each house model being chosen:
- Model A: 0.15
- Model B: 0.25
- Model C: 0.10
- Model D: 0.20
- Model E: 0.15
- Model F: 0.15
2. Define the probability of a client opting for a garage:
- Garage: 0.60
- Carport: 0.40
3. Run a simulation to generate a large number of random selections of house models and garage/carport options.
4. For each selection, record whether the client chose model B with a car garage.
5. Calculate the frequency of clients choosing model B with a car garage over the total number of simulations to approximate the probability.
Here is a simple Python code snippet to simulate this scenario:
```python
import random
# Define probabilities
house_models = ['A', 'B', 'C', 'D', 'E', 'F']
model_probabilities = [0.15, 0.25, 0.10, 0.20, 0.15, 0.15]
garage_options = ['garage', 'carport']
garage_probabilities = [0.60, 0.40]
# Simulation parameters
num_simulations = 10000
num_b_with_garage = 0
# Run simulations
for _ in range(num_simulations):
chosen_model = random.choices(house_models, weights=model_probabilities)[0]
chosen_garage = random.choices(garage_options, weights=garage_probabilities)[0]
if chosen_model == 'B' and chosen_garage == 'garage':
num_b_with_garage += 1
# Calculate probability
probability_b_with_garage = num_b_with_garage / num_simulations
print('Probability of choosing model B with a car garage:', probability_b_with_garage)
```
This code will run the simulation to generate frequencies and approximate the probability of a client choosing model B with a car garage. The more simulations you run, the more accurate the probability estimate will be.
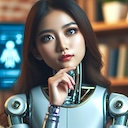
To simulate the likelihood of a client choosing model B with a car garage, follow these steps:
1. Define the probabilities for each house model and garage option.
2. Run a simulation with a large number of random selections.
3. Record how many times model B with a car garage is chosen.
4. Calculate the frequency of this selection to estimate the probability.
Use the provided Python code snippet to conduct this simulation and determine the probability accurately.
Use the results of the simulation to calculate the probability that a client
will choose model B with a car garage.
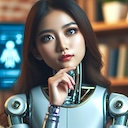
In the provided Python code snippet, the probability of a client choosing model B with a car garage is calculated at the end of the simulation. The `probability_b_with_garage` variable stores the frequency of clients choosing model B with a car garage over the total number of simulations. This probability is then printed out after the simulation runs.
By running the simulation with a large number of iterations (e.g., `num_simulations = 10000`), the calculated probability should provide a good estimate of the likelihood that a client will choose model B with a car garage based on the defined probabilities for house models and garage options.
less big words
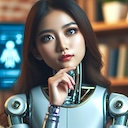